Vue Js String Split Method: The split() method in vue.js is used to split a given string into an array of substrings and returns the new array. This split function divides a string into an array of substrings by separating the string into pieces using a specified separator, which can be either a character or regular expression . The original string is unchanged by the split() method. this.string.split(separator, limit) is the syntax for Vue.js’s split() method. We will describe how to apply this method in Vuejs in this article.
How to convert a string to a string array in Vue JS?
Converting a string to a string array can be achieved by using the this.string.split() method in Vue Js
Split string into array Vue JS
<div id="app">
<button @click="myFunction">click me</button>
<p>Text: {{string}}</p>
<p>Splited String :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string :'its important to learn how to use the framework effectively ',
results:''
}
},
methods:{
myFunction(){
this.results = this.string.split(" ");
},
}
}).mount('#app')
</script>
Output of above example

How to convert a string to a list in Vue.js?
To convert a string to a list in Vue.js, you can use the split() method, which will return an array after breaking the given string by a specified separator
Vue Js Spread string into list
<div id="app">
<button @click="myFunction">click me</button>
<p>Text: {{string}}</p>
<p>List of Character :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string :'Fontawesomeicons',
results:''
}
},
methods:{
myFunction(){
this.results = this.string.split("");
},
}
}).mount('#app')
</script>
Output of above example
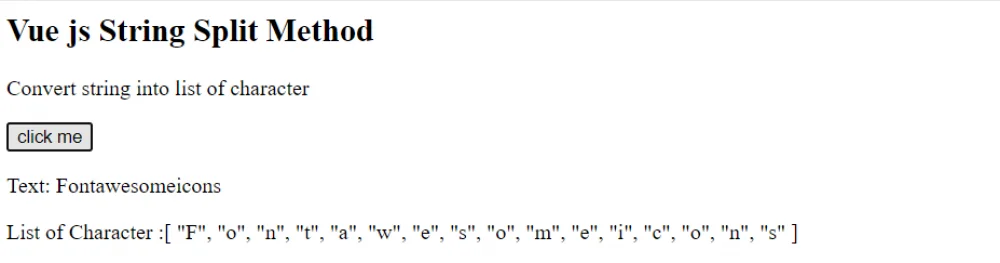